Building a mobile application with Vue.js is not impossible. In fact, you can build production-ready apps that look and feel like native mobile apps with Vue.js.
In this tutorial, we’ll show you how to develop a mobile app with Vue.js. We’ll discuss the merits of using Vue.js to build mobile applications, discuss how to choose the right Vue.js framework for your mobile project, and evaluate the overall developer experience.
Here’s what we’ll cover:
- Why use Vue.js to build mobile apps?
- Vue.js frameworks for mobile app development
- Choosing the right Vue.js mobile framework
Why use Vue.js to build mobile apps?
Below are some reasons why you should consider using Vue.js for your next mobile app development project.
Cross-platform
Cross-platform app development is about building a single application that can run on various Android and iOS operating system instead of developing different app versions for each platform. This will save you the time it would take to learn a programming language like Java for Android or Swift for iOS development. You just have to focus on the skill you already have (Vue.js).
Easy to learn
Vue.js enables you to build high-quality, production-ready Android and iOS apps with a single codebase using the skills you’re already familiar with — namely, HTML, CSS, and JavaScript.
Great documentation
Vue.js has some of the best documentation in the frontend developer community. Going through the docs feels like talking to your own personal Vue.js sensei. The excellent documentation will, of course, increase your efficiency and productivity when building mobile apps.
Vue.js frameworks for mobile app development
In no particular order, here are the top frameworks for building mobile applications with Vue.js:
Let’s take a closer look at each Vue.js mobile framework.
Ionic Vue
Ionic is an open-source mobile UI toolkit for building high-quality, cross-platform, native web app experiences and desktop apps using web technologies (HTML, CSS, and JavaScript) with integrations for popular frameworks such as Angular, React, and Vue.
Ionic is truly framework–agnostic as it allows you to use the JavaScript framework you are most comfortable with. This means you can decide to use React, Vue, Angular, Vanilla JavaScript, or even Stencil. Indeed one can say the Ionic for Everyone movement is now a success. With Ionic, you can build web, mobile (Android and iOS), PWA, and desktop applications all in one codebase.
One of the main reasons why you should consider using Ionic Vue is that it has great sets of premade UI components that make building native iOS, native Android, and web application easy and fast.
Ionic components render differently based on the platform you’re running your application on. For example, when your application is running on an Android device, the Material Design pattern is used to render UI components. That said, the shift between building web application with Vue and with native Android and iOS apps isn’t earth-shattering.
Let’s take a look at how the Ionic alert component renders differently on Android and iOS platforms in an Ionic Vue app:
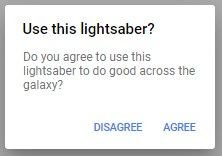
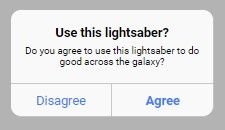
Ionic Vue was officially launched in October 2020 following the release of Ionic Vue beta in early 2019. This beta version was built for Vue 2 while the current stable version was built to support Vue 3. It is TypeScript-based, though you can change the setup of your project to work with JavaScript.
Since Vue.js is a web framework, it does not natively support mobile app development. So how do we get access to native mobile features such as the camera and geolocation? Ionic has an official native runtime called Capacitor. With Capacitor’s plugin, you can access the native API of the device your app is running on and build truly native mobile application with Ionic Vue.
Here’s how to get access to a user’s location from their device using the Capacitor Geolocation Plugin in an Ionic Vue app:
import { Plugins } from '@capacitor/core'; async getLocation() { const { Geolocation } = Plugins; const coordinates = await Geolocation.getCurrentPosition(); this.coords.lat = coordinates.coords.latitude; this.coords.lng = coordinates.coords.longitude; }
Although Ionic Vue is new, if you have basic knowledge of Vue 3, there isn’t really much of a learning curve. Building with Ionic Vue should be easy if you’re already familiar with Vue.
Framework7 Vue
Framework7 is a free and open-source framework for developing mobile, desktop, and web apps with a native look and feel. It’s also an indispensable prototyping tool.
Similar to Ionic, Framework7 enables you to build mobile apps with Vue.js, React, Svelte, or vanilla JavaScript.
Framework7 Vue offers stunning UI kits that look and feel native. To get the best out of Framework7 Vue, I recommend using its components to build the user interface of you mobile application whenever possible. For example, avoid using the normal HTML button element to create a button; instead, use the f7-button
. This will make your mobile application look and feel native and work properly when compiled down to Android and iOS.
The easiest way to get started with Framework7 Vue is to use this template so all all you have to focus on is your application logic; webpack, Cordova, Framework7 Icon, Material Design Icon, and Vue.js are already installed and configured. This template also comes with routing system as well as Vuex for state management.
It’s worth nothing that Framework7 doesn’t use Vue router; routing and navigation is done using the f7router
(Framework7 router). The API is quite similar to that of the official Vue router.
The official Framework7 Vue documentation is decent, though it is missing some key information, which can be quite frustrating.
To get access to the native APIs of mobile devices, Framework7 Vue uses Apache Cordova, a runtime that provides a wrapper for your web application built with Framework7 Vue to native Android and iOS applications. This is where the Ionic Vue team really got it right: with Capacitor as its official runtime, you don’t need to worry about Cordova falling out of date or not supporting key features.
Vue Native
Vue Native is a JavaScript framework designed to help you build cross-platform mobile applications with JavaScript. Vue Native is a wrapper around React Native that enables you to build mobile applications using Vue.js. With Vue Native, you can do everything you can do with with React Native, and your code is compiled down to React Native.
The easiest way to get started with Vue Native is to use the Vue Native CLI. The CLI generates a simple, one-page application using the Expo CLI or React Native CLI under the hood. This means you’ll have to install the Expo or React Native CLI to use the Vue Native CLI.
To install the Expo CLI globally, run the following command in your terminal:
npm install -g expo-cli
Optionally, you can generate your project with bare-bones React Native by installing the CLI:
npm install -g react-native-cli
The next step is to install the Vue Native CLI globally:
npm install -g vue-native-cli
For Expo users, run the following to generate a project with Vue Native:
vue-native init <projectName>
For React Native, use this command:
vue-native init <projectName> --no-expo
To get access to mobile device APIs, Vue Native uses packages from the Expo and React Native communities. This is a great reason to consider using Vue Native for your next project since you are already covered by the React Native community. Also, in a Vue Native project, you can make use of the NativeBase React Native component to build the UI of your mobile application.
Notice how the entire Vue Native ecosystem depends on the React Native and Expo ecosystem? The growth of Vue Native is tied to React Native, which could be a negative or positive, depending on your perspective. Relying on React Native limits the Expo team’s flexibility to innovate, but it also means the growth of Vue Native is tied to React Native, which is by far the most popular and active project for developing mobile applications with JavaScript. In addition, Vue Native allows you to use React Native libraries.
One downside to using Vue Native compared to Framework7 Vue and Ionic Vue is that you can’t run a Vue Native project in the browser. You have to install Android Studio or Xcode to get a preview of what you’re building.
Another drawback is that, unlike Framework7 Vue and Ionic Vue, Vue Native components won’t work for web development because it is a mobile framework.
NativeScript Vue
NativeScript is an open-source JavaScript framework that prides itself on helping you build truly native Android and iOS apps with JavaScript. The beauty of NativeScript is that you can build native mobile applications with using Angular, Vue.js, or Vanilla JavaScript.
NativeScript Vue is a framework that combines Vue and NativeScript in one package, allowing you to build cross-platform iOS and Android apps using Vue.
Unlike in Ionic Vue and Framework7 Vue, which enable you to use regular HTML elements, in NativeScript Vue, elements such as <div>
do not exit. To build the user interface of your app, you will have to use the NativeScript UI components.
NativeScript Playground is a tool made by NativeScript developers that enables you to tinker and see how NativeScript works. You can easily build a NativeScript Vue app with this playground by selecting the Vue template.
Choosing the right Vue.js mobile framework
Now that you’re aware of the top frameworks for building mobile applications with Vue.js, let’s consider some key factors to consider when choosing a framework for your project. This is important especially when building for production because you want to avoid picking a framework that might impose unforeseen limitations on your project after spending a lot of time writing thousands of lines of code.
Community support
Community support is one of the most important factors to consider when choosing a framework. This affects how easy it is to find answers to fix bugs in your code and, in turn, your productivity.
Let’s take a look at the metrics from GitHub to see how active each framework’s developer ecosystem is.
At the time of writing, Vue Native has 15 contributors, eight releases, 7.9k stars and it has been forked by 291 users on GitHub.

Ionic framework has 401 contributors, 284 releases on npm, 43.2k stars and it has been forked by 13.4k users on GitHub.

NativeScript Vue has 43 contributors, 95 releases, 4.6k stars, and has been forked by 224 users on GitHub.

Framework7 has 134 contributors, 222 versions on npm, 16.1k stars, and has been forked by 3.3k users on GitHub.

Another factor to consider is how long these frameworks have been around in relation to the adoption rate. Although Ionic has been around since the days of AngularJS (circa 2013), the stable version of Ionic Vue was released in October 2020 and has enjoyed a fairly swift adoption rate. As of the time of writing, it has over 6,651 weekly downloads on npm.
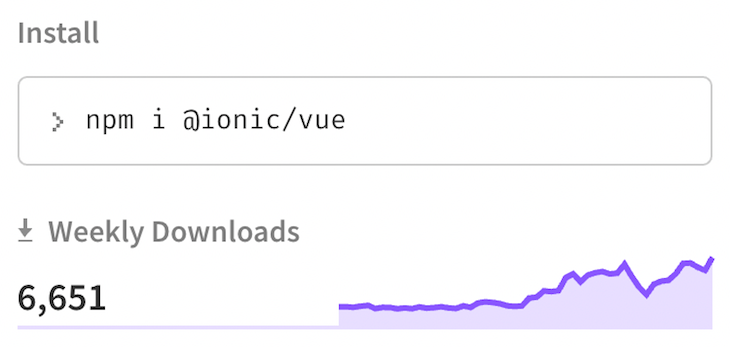
Framework7 Vue was released in 2016. Compared to Ionic Vue, the adoption rate is pretty low:
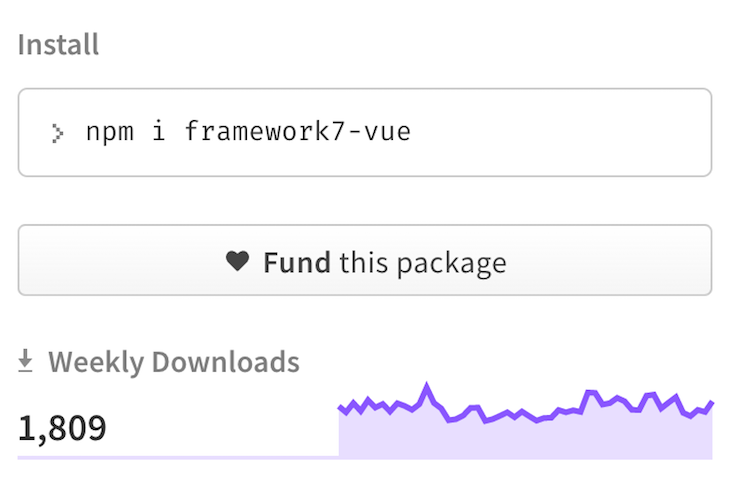
As a result, the community is comparatively small. You might find yourself sinking a lot of time into searching for answers to bugs. In fact, most of the responses to questions in the forum are from the creator himself.
The first version of Vue Native was released on Aug. 1, 2018. Again, its 601 weekly downloads pale in comparison to Ionic Vue:
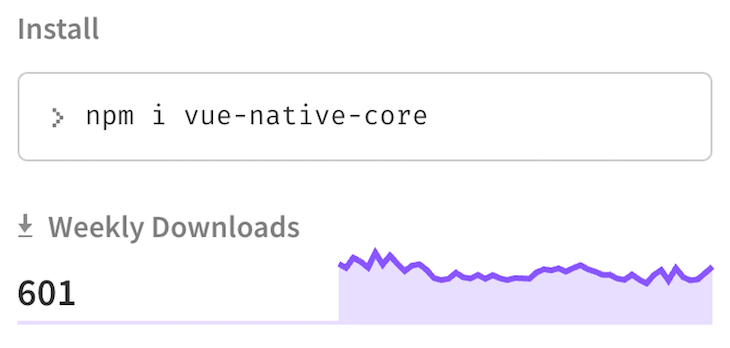
The first version of NativeScript Vue was released on July 9, 2017. Though not as popular as Ionic Vue in terms of weekly downloads, its 1,746 at the time of writing is nothing to sniff at:
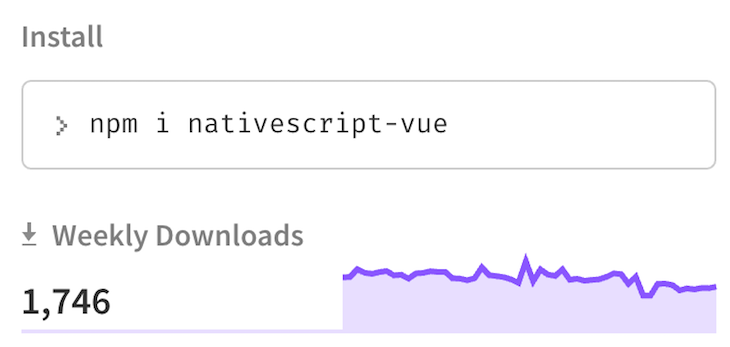
This shows developers’ interest in the framework, which reflects the activeness of the open-source community in making improvements and fixing bugs.
In case you haven’t been paying attention, if you’re going purely by the numbers, Ionic Vue is the most popular framework for Vue.js mobile app development.
Native tooling
This is an important factor to consider when building mobile applications in general. Let’s say you’re building a mobile app that features biometric authentication via fingerprint scan, for example. You’ll need to look out for a framework that can provide that tooling.
In Framework7 Vue and Ionic Vue apps, Cordova can be used to access the native APIs of the device. Ionic Vue also allows you to use Capacitor for native functionality. This means you have access to the native tooling in the Cordova and Capacitor ecosystem.
Vue Native is super valuable here because you can use Expo and React Native tools to get access to device API.
NativeScript Vue allows you to access native device APIs using NativeScript plugins. This is a big plus, especially since the NativeScript community is fairly large and active. In February 2021, NativeScript announced support for Capacitor.
UI components
Ionic Vue has more than 100 sets of beautiful, elegant UI components. The components are quite easy to use; if you have decent knowledge of Vue 3, using the components in Ionic Vue will be a walk in the part.
Framework7 Vue has tons of premade UI components, and the best part is that you can still use the core components in your Framework7 Vue mobile app. Though the implementation may be different, the core components usually work in a Vue.js app seamlessly.
NativeScript Vue has a handful of UI components. Though it doesn’t offer as many components as Ionic Vue and Framework 7 Vue, they are elegant and render differently based on device platform (Android, iOS, etc.).
Developer experience
Now let’s talk about the developer experience. We’ll consider factors such as hot reload, the ability to run apps on the web, etc.
We Vue.js developers typically run our apps in the browser and debug mostly with the browser console. In a Framework7 Vue or Ionic Vue project, you can run your mobile app on the web browser and still get platform-specific styles. Ionic also has a feature called Ionic Lab that lets you view your app to see how it will look on Android, iOS, and Windows. Vue Native, however, does not support this feature.
NativeScript has a playground you can use to develop and preview your app. By downloading the mobile app on the App Store or Google Play, you can preview your app on your device by scanning the QR code.
Documentation
The official docs for Ionic Vue are awesome. They include a preview of how your components will look on Android and iOS, and you can build your app without looking for resources outside the Ionic and Capacitor documentation.
The Framework7 Vue docs are great for the most part, but incomplete. For example, how to programmatically navigate with the f7-router
is not in the official docs.
NativeScript Vue has fairly decent documentation. Though not as elaborate as Ionic Vue, it’s still comprehensive.
The Vue Native docs are not quite as useful as the others. There aren’t many tutorials or articles online covering Vue Native, either.
Learning curve
Ionic Vue uses Vue 3, which means if you’re not used to how things work in Vue 3, you’ll have to take an extra step to learn it. Other than that, most things work normally as they would in a Vue web application.
NativeScript Vue and Vue Native will require a slight learning curve because things work quite differently from a conventional Vue.js app. For example, below is a NativeScript button with a tap (click) event:
<Button text="Button" @tap="onButtonTap" />
In a regular Vue app, the @click
event would be used.
Here is a click event in a Vue Native application:
<button :on-press="onPressLearnMore" title="Learn More" />
In Framework7 Vue, things are pretty standard and work as they would in a normal Vue.js app. Using Framework7 components in a Vue.js app is just like working with Bootstrap components. It’s pretty simple.
Conclusion
Choosing a Vue.js framework for building mobile applications depends on a lot of factors, including learning curve, application demands, ecosystem of the framework, performance, and popularity.
In this tutorial, we covered the top frameworks available for building mobile applications with Vue.js and compared the strength and weaknesses of each. At the end of the day, each Vue.js mobile app development framework is great in its own way. When deciding which framework to use in your next mobile project, check the metrics we discussed and weigh them against the unique goals and requirements of your app.
The post Comparing Vue.js mobile app development frameworks appeared first on LogRocket Blog.